Universal Data Access Components (UniDAC) is a powerful library of non-visual components that provides direct access to multiple databases from Delphi, C++Builder, and Lazarus, including Community Edition, on various platforms such as Windows, Linux, macOS, iOS, and Android. This cross-database data access solution is designed to simplify the process of developing database-related applications and increase their performance and adaptability.
UniDAC offers a unified, server-independent interface for working with different databases, allowing developers to switch between various database servers in their cross-database UniDAC-based applications by merely changing a single connection option. This flexibility makes UniDAC an excellent tool for creating scalable, server-independent applications.
Performance tuning, on the other hand, is a critical aspect of database application development. It involves optimizing the speed and efficiency of database operations, which is crucial for applications that handle large amounts of data or have many concurrent users. Effective performance tuning can significantly improve the user experience by reducing wait times for database operations, making applications more responsive, and enabling them to handle larger workloads.
With UniDAC, performance tuning becomes a more straightforward process. Its server-aware providers ensure the best way to perform operations specific to the server, and its advanced data access algorithms and optimization techniques are designed to write high-performance, lightweight data access layers. By understanding and effectively leveraging these features, developers can significantly enhance the performance of their UniDAC-based applications.
In the following sections, we will delve deeper into the architecture of UniDAC, its key features, and how to leverage them for optimal database access and performance tuning.
Understanding UniDAC Architecture
The architecture of Universal Data Access Components (UniDAC) is designed to provide a unified, server-independent interface for working with different databases. This architecture is built around several key components and supports a wide range of Database Management Systems (DBMSs), enabling universal data access.
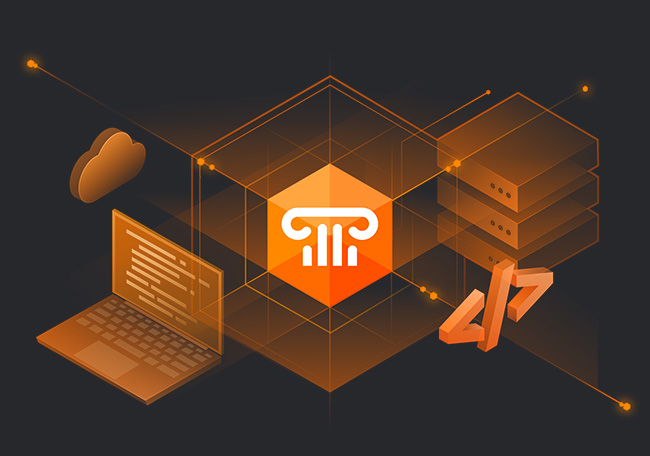
UniDAC Components
UniDAC is composed of several components that work together to provide direct access to multiple databases. These components include server-specific data providers that ensure the best way to perform operations on the server, a core engine that provides a common API for database operations, and a set of data access components that abstract the specifics of each database server. These components work together to provide a unified, server-independent interface for database operations.
Supported Database Management Systems (DBMSs)
UniDAC supports a wide range of DBMSs, allowing developers to work with multiple databases using a single interface. The supported DBMSs include Oracle, SQL Server, MySQL, PostgreSQL, SQLite, and many others. In addition to these traditional relational databases, UniDAC also supports NoSQL databases like MongoDB and cloud services such as Salesforce, QuickBooks, and others. This wide range of supported DBMSs makes UniDAC a versatile tool for developing database applications.
UniDAC’s Universal Data Access
One of the key features of UniDAC is its universal data access. This feature provides a transparent, server-independent interface for working with different databases. With universal data access, developers can easily switch between different database servers in their UniDAC-based application by changing a single connection option. This means that a UniDAC-based application can work with Oracle, switch to MySQL, and then to PostgreSQL, all without significant code changes. This flexibility makes UniDAC an excellent tool for developing scalable, server-independent applications.
In conclusion, the architecture of UniDAC is designed to provide a flexible, efficient, and unified interface for working with multiple databases. Its key components and wide range of supported DBMSs enable universal data access, making it a powerful tool for developing database applications. In the following sections, we will explore how to set up and manage database connections with UniDAC, execute SQL commands, work with UniDAC dataset classes, and more.
Setting Up and Managing Database Connections with UniDAC
Creating and managing database connections is a crucial part of any database application. With UniDAC, this process is simplified and streamlined. Here are step-by-step instructions on how to set up database drivers and manage database connections with UniDAC.
Setting Up Database Drivers
- Install UniDAC: Before setting up database drivers, ensure that UniDAC is installed in your development environment. You can download it from the official Devart website and follow the installation instructions.
- Choose the Database Provider: UniDAC supports a wide range of databases. Choose the provider corresponding to the database you are using. For example, if you’re using Oracle, you would choose the Oracle provider.
- Configure the Provider: Each provider has a set of properties that you can configure to suit your needs. These properties include the database name, username, password, and others. Configure these properties according to your database setup.
Managing Database Connections
Create a Connection: To create a connection, instantiate the TUniConnection component. This component represents a connection to a database.
var
UniConnection: TUniConnection;
begin
UniConnection := TUniConnection.Create(nil);
end;
Set Connection Properties: Set the properties of the TUniConnection component to match your database setup. The ProviderName property should match the database provider you’re using. The Username, Password, and Database properties should match your database credentials.
begin
UniConnection.ProviderName := 'Oracle';
UniConnection.Username := 'myUsername';
UniConnection.Password := 'myPassword';
UniConnection.Database := 'myDatabase';
end;
Open the Connection: Once the connection properties are set, you can open the connection using the Open method.
begin
UniConnection.Open;
end;
Use the Connection: With the connection open, you can now use it to execute SQL commands, work with datasets, and perform other database operations.
Close the Connection: When you’re done with the database operations, close the connection using the Close method.
begin
UniConnection.Close;
end;
Destroy the Connection: Finally, when the connection is no longer needed, destroy it to free up resources.
begin
UniConnection.Free;
end;
Remember, managing database connections effectively is crucial for the performance and reliability of your application. Always close connections when they’re no longer needed, and handle any connection errors appropriately to ensure your application remains robust and responsive.
Working with UniDAC Dataset Classes
Working with datasets is a fundamental part of database programming. UniDAC provides a set of dataset classes that make it easy to retrieve, navigate, and manipulate data. In this section, we’ll cover how to edit dataset content, use UniDAC to edit dataset data, and post changes to a database.
Editing Dataset Content
Retrieve the Dataset: The first step in editing dataset content is to retrieve the dataset. You can do this by executing a SQL SELECT statement using the TUniQuery component.
var
UniQuery: TUniQuery;
begin
UniQuery := TUniQuery.Create(nil);
UniQuery.Connection := UniConnection;
UniQuery.SQL.Text := 'SELECT * FROM myTable';
UniQuery.Open;
end;
Navigate the Dataset: Once you have the dataset, you can navigate it using the First, Next, Previous, and Last methods. For example, to move to the next record, you would use the Next method.
begin
UniQuery.Next;
End;
Using UniDAC to Edit Dataset Data
Start the Edit: To edit the data in a dataset, you first need to start the edit operation. You can do this using the Edit method.
begin
UniQuery.Edit;
end;
Modify the Data: Once the edit operation has started, you can modify the data in the dataset. You can access the fields of the current record using the FieldByName method.
begin
UniQuery.FieldByName('myField').AsString := 'New Value';
end;
Posting Changes to a Database
Post the Changes: After you’ve made the necessary changes to the dataset, you need to post the changes back to the database. You can do this using the Post method.
begin
UniQuery.Post;
end;
Check for Errors: When posting changes to the database, it’s important to check for any errors that might occur. You can do this using a try/except block.
begin
try
UniQuery.Post;
except
on E: EUniError do
ShowMessage('An error occurred: ' + E.Message);
end;
end;
Close the Dataset: Once you’re done with the dataset, you should close it to free up resources.
begin
UniQuery.Close;
end;
By understanding and effectively using UniDAC’s dataset classes, you can easily retrieve, navigate, and manipulate data in your database applications. In the following sections, we’ll delve deeper into other aspects of UniDAC, such as working with database metadata and preparing a UniDAC application for runtime.
Performance Tuning Techniques in UniDAC
Performance tuning is a critical aspect of database application development. It involves optimizing the speed and efficiency of database operations, which is crucial for applications that handle large amounts of data or have many concurrent users. UniDAC provides several features and techniques that can be used to enhance the performance of your database applications.
Direct Mode
Direct Mode is a feature of UniDAC that allows your application to work with a database directly without involving a database client library. This significantly simplifies the deployment and configuration of your applications and can also improve performance by reducing the overhead associated with client libraries.
To enable Direct Mode, set the Direct property of your TUniConnection component to True.
begin
UniConnection.Direct := True;
end;
Mobile Development
UniDAC provides full support for mobile development, allowing your mobile applications to work with multiple databases as easily as desktop applications do. This can significantly improve the performance of your mobile applications by enabling them to directly access and manipulate database data without the need for a separate server-side API.
Server-Independent SQL
UniDAC provides a powerful macros engine that supports server-independent SQL. This allows you to write SQL queries that work with multiple databases, regardless of their specific SQL dialects. By using server-independent SQL, you can avoid the need to write and maintain separate queries for each database, which can significantly improve the maintainability and performance of your application.
Access Cloud Services
UniDAC provides the ability to work with data stored in various cloud services, such as Salesforce, QuickBooks, Zoho CRM, and others. This is achieved through the UniDAC ODBC provider in conjunction with Devart ODBC drivers for Clouds. By accessing cloud services directly from your application, you can improve performance by reducing the need for intermediate APIs or services.
Secure Connection
UniDAC allows you to establish a secure connection to your database server using SSL, SSH, or HTTP/HTTPS protocols. This not only enhances the security of your application but can also improve performance by reducing the risk of network-related issues or attacks that could slow down your application.
To establish a secure connection, you can use the SSLOptions property of your TUniConnection component.
begin
UniConnection.SSLOptions.Mode := sslmUnspecified;
UniConnection.SSLOptions.Cert := 'myCert.pem';
UniConnection.SSLOptions.Key := 'myKey.pem';
UniConnection.SSLOptions.CA := 'myCA.pem';
end;
By leveraging these features and techniques, you can significantly enhance the performance of your UniDAC-based applications. In the next section, we’ll discuss how to debug UniDAC applications and handle any errors that might occur.
Conclusion
Throughout this guide, we’ve explored the various aspects of UniDAC, from setting up and managing database connections to working with dataset classes and performance tuning techniques. The goal has been to provide a comprehensive understanding of how to leverage UniDAC for optimal database access.
We’ve discussed several performance tuning techniques, including the use of Direct Mode, mobile development, server-independent SQL, accessing cloud services, and establishing secure connections. These techniques, when properly implemented, can significantly enhance the performance of your UniDAC-based applications, making them more responsive and capable of handling larger workloads.
UniDAC offers a host of benefits for database access. Its universal data access allows you to work with multiple databases using a single interface, simplifying the development process and increasing the adaptability of your applications. Its support for a wide range of DBMSs and cloud services makes it a versatile tool for developing database applications. Furthermore, its advanced features like Direct Mode and server-independent SQL can significantly improve the performance and maintainability of your applications.
In conclusion, UniDAC is a powerful tool for database application development. Its flexible architecture, wide range of supported DBMSs, and advanced features make it an excellent choice for developers seeking to create robust, high-performance database applications. By understanding and effectively leveraging the features and techniques discussed in this guide, you can make the most of UniDAC and create applications that provide optimal database access.